Nested If statement issues
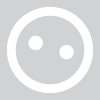
Is there a good way to do a true "nested" if statement in the game? The issue I'm running into is something like this:
If Game.BoolTest = True AND If Game.IntTest > 0
If Game.IntTest = 1
Then STUFF
If Game.IntTest = 2
Then OTHER STUFF
If Game.IntTest = 3
Then etc etc
If I implement something like that... the game checks for the first 2 conditions to be met, then it threads all other If statements below. After that, only the bottom If statement requirements appear to be necessary... So for instance, OTHER STUFF will activate if IntTest = 2 but if BoolTest = False.
At the best, I have to create a whole bunch of separate statements redundant statements to work around this... but at the worst its driving me insane trying to come up with workarounds that don't require making an insane amount of extra variables for each actor. Thoughts?
If Game.BoolTest = True AND If Game.IntTest > 0
If Game.IntTest = 1
Then STUFF
If Game.IntTest = 2
Then OTHER STUFF
If Game.IntTest = 3
Then etc etc
If I implement something like that... the game checks for the first 2 conditions to be met, then it threads all other If statements below. After that, only the bottom If statement requirements appear to be necessary... So for instance, OTHER STUFF will activate if IntTest = 2 but if BoolTest = False.
At the best, I have to create a whole bunch of separate statements redundant statements to work around this... but at the worst its driving me insane trying to come up with workarounds that don't require making an insane amount of extra variables for each actor. Thoughts?
Comments
when game.IntTest ≠ 0 instead is >0
because all the other when statements are active; they all are >0
you code tells the computer to do all of them at once...
and, attribute type on those conditions...should be an index.
MH
When Game.BoolTest = True
When Game.IntTest = 1
Then STUFF
When Game.IntTest = 2
Then OTHER STUFF
When Game.IntTest = 3
Then etc etc
Providing the 1,2 and 3 whens (ifs) are nested (not with each other) but in the first "When Game.BoolTest is True" you should have no problems.
Finally, the otherwise sections work well with booleans, so if game.BoolTest is false, you don't have to put when game.BoolTest is false in the otherwise section, just put the stuff to do, if you need to.
:-)
""You are in a maze of twisty passages, all alike." - Zork temp domain http://spidergriffin.wix.com/alphaghostapps
If you're interested in more accurate pseudocode, this is a statement I'm currently using that works.
If Game.Item1 != Game.Item1.Current (If the item state has changed)
AND
If Game.Item1 > 0 (If the item isn't -1 (deleted state) or 0 (not spawned state))
AND
If self.position.X = Game.Slot1.X (If the current position of the item is in "slot1")
THEN
Blah Blah
So now, pretend that there are 10 Game.Slots. That means, how I have it currently implemented, I'd have to copy this function 10 times, for every item in the game! And since I can't seem to find a find/replace feature (not even in the XML files since variable names are referenced as number sequences), this is quite an annoying task.
This is how I originally tried it:
If Game.Item1 != Game.Item1.Current
AND
If Game.Item1 > 0
THEN
If self.position.X = Game.Slot1.X (nested if)
THEN
Blah Blah
If self.position.X = Game.Slot2.X (nested if)
THEN
Blah Blah
etc etc
So in this case, I only have a handful of references to change when I copy this to a new item, not to mention it must be more efficient. Problem is, once the bottom If statements get threaded, only the bottom condition is required (if self.position.X = Game.Slot#.X). So as you can imagine, an item would go flying through the slots unchecked after it happens the first time. Yes, there are workarounds using bools, but that requires just as much work to create a new bool for every statement.
Gyroscope, from my experimentation it seems the example you provided wouldn't work. Game.IntTest would keep firing regardless of whether the boolean remained true or false. That's the core of my issue here.
One thing I will say, the example I made based on your original pseudocode didn't work with When mouse is down for some reason, only When touch is pressed.
""You are in a maze of twisty passages, all alike." - Zork temp domain http://spidergriffin.wix.com/alphaghostapps
I could have fixed that with a boolean for each and every item... one wouldn't have done since multiple items are moving simultaneously. Instead I realized I needed some random Self.Value to use. So I chose to say "If Self.Density = 0" and then immediately I set Self.Density to 0.1. Then after all the If statements I wait like 0.1 seconds then change Self.Density back to 0 so further activations will work. Really jacked up logically but it works, lol.
when *something* and self.DoneCalc = 0
- do *something* and change self.DoneCalc to self.DoneCalc +1
- when *something* and self.DoneCalc = 1
-- do *something* and change self.DoneCalc to self.DoneCalc +1
-- when *something* and self.DoneCalc = 2
--- do *something* and change self.DoneCalc to self.DoneCalc +1