Indexes, how do they work
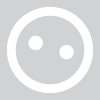
So for my game I need to make a Hex Grid which is turning out to be really difficult in GS since the actor parameters in editor all assume basically a cubic shape, but my life would be much improved if I could superimpose a index-based coordinate system on the whole shebang, trouble is, I'm not sure how to operate indexes in GS, it appears as if it is just another integer attribute so yeah; any help out there?
Comments
Boolean - true/false values.
Text - text values. e.g. This is a text value
Integer - Positive and negative whole numbers. e.g. 42,-42
Real - Positive and negative numbers which can have decimal numbers. e.g. 3.14159,-3.24159
Angle - Positive whole numbers between and including 0 and 359.
Index - Positive whole numbers greater or equal to 0. e.g 0 and anything greater
Then, have something happen everytime you click one of these actors... set Game.Current.Index.X = Self.Index.X and Game.Current.Index.Y = Self.Index.Y. Then the game knows exactly where the player touched and can respond accordingly.
That wouldn't support multi-touch too well, but then again I don't know exactly what you're trying to do with this, lol.
Starting at x-80 and y-280 just as an example. Grid size is: 160X160px
pseudo code:
game.gridsize = 40;
code for tiles on even lines:
(local boolean)
even = true;
x positioning:
if this._x >= 80 && this._x <= 240
then ceil((this._x/game.gridsize)*game.gridsize)
y positioning:
if this._y >= 120 && this._y <= 280
then ceil((this._y/game.gridsize)*game.gridsize)
if even == false
x positioning:
if this._x >= 100 && this._x <= 220
then (ceil((this._x/game.gridsize)*game.gridsize)) + gridsize/2
y positioning:
if this._y >= 140 && this._y <= 260
then (ceil((this._y/game.gridsize)*game.gridsize)) + gridsize/2
@FreshlyMinted
I would say you need 3 indexes...
1. HexPicked
2. HexX
3. HexY
a controller off-screen Actor
with [Rules] when HexPicked = #
changeAttribute HexX to: correct #
changeAttribute HexY to: correct #
then make the size of the Hex area you want as an image (all the possibilities in that image)
(it is a good idea to have a small 5x5 colored block in the center of each hex)
for each Row the Y will be constant
for each Column in alternating rows the X will be constant.
in a new project import that image
create a crossHairs Actor
create to Display Text Actors.... one for Devices>Mouse.Position.X and one for Y
create an Actor and add a note to it...numbering the hexes from left to right (1 to whatever)
Preview and write down each X, Y of each hex
then in your real project: (this is for drag-able actors... )
Actor [Rule] All: when touch is released; when self.Position.X > ; when self.Position.X < ; when self.Position.Y > ; when self.Position.Y <
changeAttribute HexPicked To:
changeAttribute self.Position.X To: HexX
changeAttribute self.PositionY to HexY
for each Hex in your grid you will have to have the correct selectable area and write that rule.
but you only need to do that once on one actor... then drag the rule to myBehaviors and drag to the other Actors...
now that your are totally confused... you can try it out and try out a way for the game to control grid placement.
so many ways to skin this cat! ;D
MH
Let's say you'd like to make a grid of 20x20 square, each 32x32.
That means that, if mouse is at 64x 0y, it's in the second square. Now, if you take the 64x, and divide it by the grid width (32), you got 2. What if mouse is at...50? 50/32 = 1,56, and that's not a valid square. Just ceil it
So basically
2 variables, tileWidth, tileHeight = 32, 32;
if mouse is released (this way the check happens when touch is released, but you can set it up for when it's down too, just use constrain instead of change attribute to keep checking)
change attribute game.squareCoordX = ceil(mouse.x/tileWidth)
change attribute game.squareCoordY = ceil(mouse.y/tileHeight)
And you got the square indexes. Now, to use them to put an actor right in the middle of a block, you just have to multiply back those values. if game.squareCoordX is 2, and game.squareCoordY is 1, you multiply them by tileWidth and tileHeight.
newPositionY = game.squareCoordY(1)*tileHeight(32) = 32.
newPositionX = game.squareCoordX(2)*tileWidth(32) = 64.
note that that's not the middle of the square, you still have to do subtract to that, half of the width of the tile. 64-16 = 48. That's the x center of the square 2,1.
then subtract half of the tile height to game.squareCoordY = 32-16 = 16
The new coordinate that the object will have are 48 for the x, and 16 for the y. Exactly at square 2,1.
This should work flawlessly, but I came up with it now, so I can't guarantee :P Obviously, you'll have to draw the grid lines. It just lets you go without having an actor for each square (bad) or lots of if statement (kinda sloppy).
That wasn't sarcasm was it? :P It's just some simple if statements or "rules" to snap pieces to specific points. Any gs user should be able to follow it. Also the last one was supposed to be:
if this._y >= 140 && this._y <= 260
then (ceil((this._y/game.gridsize)*game.gridsize)) - game.gridsize/2
not "+ game.gridsize/2"
If you still want to index, like I said you can base your index off of dividing their position by grid width or screen width or from the center.