Turn based games with computer opponents
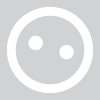
Hi, I'm new to this GS stuff - not a programmer, although I've programmed basic Flash - self taught. I'd like to do some turn based games using a computer opponent on either square or hex grids where actors slide to and snap to centres of cells. Have there been examples of this type of game done on GS, like checkers, othello and Hex? Is GS the best platform to use, or are there other solutions?
Comments
I'd suggest starting with something easier.
I don't know much about other game engines, but others might be better for this, but probably not easier to use.
Asymptoteell
It might mean using invisible actors and tons of relationships between distances between pieces and outcomes from the computer.
Artificial intelligence is tough, and you'll probably need an understanding of how gamesalad works before you'd be able to do it correctly.
More than advanced rules, it would be mostly just lots of rules and attributes.
Good luck with any game creation.
Asymptoteell
P.S. Just because AI is tough to do doesn't mean it's impossible, and it also doesn't mean most things in Gamesalad are very difficult.
MUCH easier than code.
In your example, grid squares, that's really a matrix, which is a 2D array. The computer AI would need to evaluate every square, in order to do that a loop would traditionally be used in most programming languages. GameSalad can do that but it requires a LOT more work than would be worth the effort. It CAN be done though. I wrote a post about it a long time ago. Time Slicing.
his is how I would do it, should work with little math, no actors at all. Just the mouse and a when mouse is down behaviour.
Let's say you'd like to make a grid of 20x20 square, each 32x32.
That means that, if mouse is at 64x 0y, it's in the second square. Now, if you take the 64x, and divide it by the grid width (32), you got 2. What if mouse is at...50? 50/32 = 1,56, and that's not a valid square. Just ceil it
So basically
2 variables, tileWidth, tileHeight = 32, 32;
if mouse is released (this way the check happens when touch is released, but you can set it up for when it's down too, just use constrain instead of change attribute to keep checking)
change attribute game.squareCoordX = ceil(mouse.x/tileWidth)
change attribute game.squareCoordY = ceil(mouse.y/tileHeight)
And you got the square indexes. Now, to use them to put an actor right in the middle of a block, you just have to multiply back those values. if game.squareCoordX is 2, and game.squareCoordY is 1, you multiply them by tileWidth and tileHeight.
newPositionY = game.squareCoordY(1)*tileHeight(32) = 32.
newPositionX = game.squareCoordX(2)*tileWidth(32) = 64.
note that that's not the middle of the square, you still have to do subtract to that, half of the width of the tile. 64-16 = 48. That's the x center of the square 2,1.
then subtract half of the tile height to game.squareCoordY = 32-16 = 16
The new coordinate that the object will have are 48 for the x, and 16 for the y. Exactly at square 2,1.
This should work flawlessly, but I came up with it now, so I can't guarantee :P Obviously, you'll have to draw the grid lines. It just lets you go without having an actor for each square (bad) or lots of if statement (kinda sloppy).
Should at least let you started on the grid thing :P