Spawning and destroying actors
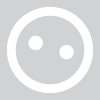
Hi,
I have a large play area in a scrolling maze-type game. I am spawning actors at various given positions, but I DON'T want them to live if they are, say, 1.5+ screens away: I only want actors to start spawning when my character gets within a given distance (so they spawn just off screen) and equally, if I manage to get away from the actor they are NOT destroyed if they JUST leave the edge of screen but ARE destroyed if I manage to get, say, 1.5 screens away.
The suggestions of checking whether an actor's self.position is < > the screen size doesn't work as the play area is many screens.
What is the correct way to do this?
Thanks!
I have a large play area in a scrolling maze-type game. I am spawning actors at various given positions, but I DON'T want them to live if they are, say, 1.5+ screens away: I only want actors to start spawning when my character gets within a given distance (so they spawn just off screen) and equally, if I manage to get away from the actor they are NOT destroyed if they JUST leave the edge of screen but ARE destroyed if I manage to get, say, 1.5 screens away.
The suggestions of checking whether an actor's self.position is < > the screen size doesn't work as the play area is many screens.
What is the correct way to do this?
Thanks!
Comments
I would suggest having two global game attributes (real) called PlayerX and PlayerY.
Within the main player actor, use two Constrain Attributes to set game.PlayerX to self.PositionX and game.PlayerY to self.PositionY
Then, in whatever actor is spawning the enemies, do something like this:
First, pick a random spawn location.
When abs(self.spawnlocationX - game.PlayerX) < 720
AND
When abs(self.spawnlocationY - game.PlayerY) < 480
Spawn the new Actor at the spawnLocationX and spawnLocationY
OTHERWISE
Pick a new spawn location...
720 is 1.5 screens in the X direction, and 480 is 1.5 screens in the Y direction.
the "abs" math function always return a positive number ( if the result is negative, it will reverse it)
You'll also have to wrap this within a Timer that acts sort of like a while loop, if
you definitely need to spawn an actor each time.
Let me know if you need help with that as well.
Something like this should do the trick.
Hope this helps!
Joe
RULE
When ANY conditions are valid
Attribute self.PositionX > (game.Character Position X)+480
Attribute self.PositionX < (game.Character Position X)-480
Attribute self.PositionY > (game.Character Position X)+320
Attribute self.PositionY < (game.Character Position X)-320
DESTROY
...so when an enemy is within range it can spawn, but when it is outside of the character's position + or - 480,320 in any direction then it is destroyed. This seems to work well as you can run a little way from an enemy so it goes off screen but if you stop it come back, but if you keep going so it leaves the specified area it dies.
Thanks for the help, it prompted me to figure this method out!