How can I determine if actor1 (circle) is completely inside actor2 (square/box)
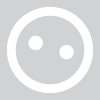
I have 2 actors:
Actor1 = "circle"
Actor2 = "square"
The first level I'm trying to make has a large square and small circle. Levels will get progressively harder where the square gets smaller.
How can I determine if the circle is completely inside the square? Just touching the edge of the square (a normal "collision") is not enough -- the user needs to get the ball completely inside the square.
Thanks!
Actor1 = "circle"
Actor2 = "square"
The first level I'm trying to make has a large square and small circle. Levels will get progressively harder where the square gets smaller.
How can I determine if the circle is completely inside the square? Just touching the edge of the square (a normal "collision") is not enough -- the user needs to get the ball completely inside the square.
Thanks!
Comments
Now in the circle's behaviors we do some checking:
if abs(circleX-squareX) <= (abs(squareSize-circleSize)/2)
and
if abs(circleY-squareY) <= (abs(squareSize-circleSize)/2)
...
do something
Now gamesalad makes this extra difficult because we can only compare an attribute to an expression, we can't compare two expressions. So we would make two attributes in the circle actor:
distanceDiffX (double)
distanceDiffY(double)
now, in the behaviors for the circle, add two rules:
constrain self.distanceDiffX to abs(self.Position.X-game.squareX)
constrain self.distanceDiffY to abs(self.Position.Y-game.squareY)
and you would now just need to compare those attributes to the sizes of the square and circle. (self.width and game.squareSize above)
I think this is accurate, but I haven't tested the math, so there could be a flaw to my logic. I'll let someone correct me if I've made a mistake, but the general concept should work.
Dave
In the square actor:
change attribute game.squareSize to self.size.width
change attribute game.squareY to self.position.Y
change attribute game.squareX to self.posiiton.X
In the circle.
Rule (Any): if self.position.X < (game.SquareX-((.5*game.SquareSize)+(.5*self.size.width)))
if self.position.X > (game.SquareX+((.5*game.SquareSize)+(.5*self.size.width)))
if self.position.Y > (game.SquareY+((.5*game.SquareSize)+(.5*self.size.height)))
if self.position.X < (game.SquareY-((.5*game.SquareSize)+(.5*self.size.height)))
change game.gameover to 1
Then wrap all your movement rules in a rule that says "when game.gameover is false"
Send and Receive Data using your own Server Tutorial! | Vote for A Long Way Home on Steam Greenlight! | Ten Years Left
Lump Apps and My Assets
If you have it set to "any" it should work.
so you could have
magnitude(abs(smallCircle.X-target.X), abs(smallCircle.Y-target.Y))
this will give you the positive (absolute value) distance between the center point of the small circle, and the center point of the target.
so if you're targer has a diameter of 100px, and the small circle has a diameter of 40. you want the magnitude to be less than 30px. (100px would be a 50px radius)
Send and Receive Data using your own Server Tutorial! | Vote for A Long Way Home on Steam Greenlight! | Ten Years Left
im using the "angry birds" example template for to create the motion... so smallCircle has a velocity...
what's the most efficient way to check it's current position when using any of the solutions here? not sure how to effectively check the rule set that scitunes explained above