[FIXED] - Mathematical Array System - [EXAMPLE]
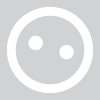
Hey,
I've just finished making a maths formula that might be useful for a lot of you.
Basically it lets you store multiple numbers in 1 integer, kind of like an array system.
Example Here : http://gamesalad.com/game/play/43213
============================ How It Works ========================================
The Array holds positions which can be filled with values (must be under the max)
Like this
| P0 | P1 | P2 | P3 | P4 | P5 |
In reality it looks like like this.
| 0 | 0 | 0 | 0 | 0 | 0 |
Note that the first position is Position 0
============================== Formulas =========================================
Adding To A Position=
x + z * ( y ^ n )
x = Array Number To Add To
z = Amount To Add To The Position
y = Max Size Of The Numbers In Positions (eg. If y=10, if positions are any higher than 9 it will go wrong)
n = Position To Add To
Extracting A Position=
floor ( x / y ^ n) % y
x = Array Number To Extract From
y = Max Size Of The Numbers In Positions (eg. If y=10, if positions are any higher than 9 it will go wrong)
n = Position To Extract
=================================================================================
What happens if position gets higher than the max?
The position will go to 0 and the next position will get 1 added to it
=================================================================================
Hope this helps you.
I'm sorry if I've got anything wrong, I'm not the best at maths.
If you have any questions please ask.
Thanks,
Vmlweb
I've just finished making a maths formula that might be useful for a lot of you.
Basically it lets you store multiple numbers in 1 integer, kind of like an array system.
Example Here : http://gamesalad.com/game/play/43213
============================ How It Works ========================================
The Array holds positions which can be filled with values (must be under the max)
Like this
| P0 | P1 | P2 | P3 | P4 | P5 |
In reality it looks like like this.
| 0 | 0 | 0 | 0 | 0 | 0 |
Note that the first position is Position 0
============================== Formulas =========================================
Adding To A Position=
x + z * ( y ^ n )
x = Array Number To Add To
z = Amount To Add To The Position
y = Max Size Of The Numbers In Positions (eg. If y=10, if positions are any higher than 9 it will go wrong)
n = Position To Add To
Extracting A Position=
floor ( x / y ^ n) % y
x = Array Number To Extract From
y = Max Size Of The Numbers In Positions (eg. If y=10, if positions are any higher than 9 it will go wrong)
n = Position To Extract
=================================================================================
What happens if position gets higher than the max?
The position will go to 0 and the next position will get 1 added to it
=================================================================================
Hope this helps you.
I'm sorry if I've got anything wrong, I'm not the best at maths.
If you have any questions please ask.
Thanks,
Vmlweb
Comments
`
High Score Position Score
1 4,321
2 3,222
3 2,999
4 1,000
5 1,000
6 570
7 0
8 0
9 0
10 0
`
If so, awesome!
I don't think you manage to fit numbers like 1000 into this unless there is a way of increasing the number of digits a integer can hold.
In my example I did some testing and once it the max number got high it started to go wrong (because of the integer size limit)
So, if you store Y positions, it would seem you have a max value for Z of about MAXINT/Y (or thereabouts) - I did not verify this definitively but mathematically it seems correct.
So my leaderboard example above should work with a Y value of 11 (if undestand what you are trying to do and the equations are correct).
MaxInt divided by the number of positions you have
note: in my formulas above Y is not the number of positions, it is the max number that can be stored in 1 position
"y = Max Size Of The Numbers In Positions (eg. If y=10, if positions are any higher than 9 it will go wrong)"
from:
"y = Max Size Of The Position Numbers (eg. for a max of 10 in a position y would be 11)"
so that does make it more clear and sound more plausible.
Would the equation to encode then be:
`
x + z + (MaxInt / y) * n ( ' * n' instead of ' ^ n')
`
Where:
`
x - the current value of the storage int (i.e. with nothing stored, it is zero)
z - is the number you want to store and is limited to 0 to MaxInt/y
y - the number of high score positions you want to be able to store in the integer
n - the position you want to store the value 'z' in (i.e. the high score slot)
MaxInt - the maximum value an integer can store
`
Notes: The higher the number 'y' the lower the range of numbers 'z' can be and thusly your high score values would be more limited in their value (and not quantity since that is defined by 'y'). You may also want to test/verify since "MaxInt / y" can yield some fractionals and thus the use of floor().
I may have my equation wrong as I was just testing with various 'y' values and 'MaxInt' at "100".
2^64/2-1
div 2 because everything in gamesalad is signed so you looose half available address space.
Codemonkey or one of the GS team could you pls tell us the max a integer can store.
I don't have a job yet cause im still at school but when I get older I wanna be a self employed iPhone game developer.
Well I suppose I kinda already am one.
If you want to see what the actual limit is just make an int and DisplayText on it and add 100,000 to it every microsecond. Eventually when it reaches 2^32/2-1 it will wrap and start counting down.
However, as I said before, I believe the upper limit on reals is a 64bit address space. I could be wrong though I haven't tested it yet.
just for example:
Log x (MaxInt)=NumOfPositions
Where x is the number of digits in a position
I was trying to associate it to bit field flags/switches stored within a single integer...which we know works and which ORBZ was thinking with his binary/shift operator reference. However, my thinking was flawed a bit and your " ^ n" is likely (nearly) correct to get the proper offsets into the number positions (but I think that is limiting the range of numbers you can store quite a bit).
The reason I say "nearly" brings me back to your demo. If you run it and then click on the bottom-most slot once, then click on the slot above it once, and then repeat that as you go upward (basically just storing "1" in each position), you will see it breaks down after you have done this to about the fifth slot or so of the lower slots. For me, it ended up populating a "19" in one of the slots above that I had not even touched yet. You can repeat the procedure starting at the second to the last/bottom one working up and you will get a "16" eventually in one of the upper (I think the top most) slots. I'm guessing each slot's logic is the same except for the power of the value used.
`2,147,483,647`
Since the first digit (i.e. the "2") can only be 0, 1, or 2, it will need to be ignored and left at zero. You could safely use the nine lowest order digits/places of the number.
If you group them into fours, you could stuff two slots/positions and each would have a range of 0 to 9999. If you group them into threes, you could stuff three slots/positions into it and each would have a range of 0 to 999. If you group them into twos, you could stuff four slots/positions into it and each would have the range of 0 to 99. Finally, if you just want to keep tracks of some lame-ass lowly high scores, you could stuff nine into them and each would have a range of 0 to 9.
Sort of chincy but was just throwing this out there for folks who maybe only want a limited but still compressed leaderboard.