Need help with simple AI for enemies
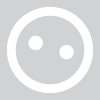
Hello all,
So, my problem is how do I make a simple AI? I've already created an updater rule that gets my main characters x and y coordinates its just that how would I get the enemy to follow him? I've tried interpolating, and moving and sometimes they would fly in the air, I need a simple Ai for my enemy that follows my main character on the ground after my character gets near the enemy.
So, my problem is how do I make a simple AI? I've already created an updater rule that gets my main characters x and y coordinates its just that how would I get the enemy to follow him? I've tried interpolating, and moving and sometimes they would fly in the air, I need a simple Ai for my enemy that follows my main character on the ground after my character gets near the enemy.
Comments
http://gamesalad.com/wiki/how_tos:gsc_follow_another_actor
:-)
""You are in a maze of twisty passages, all alike." - Zork temp domain http://spidergriffin.wix.com/alphaghostapps
I would do it like this:
First, you need to keep track of the Player's X and Y coordinates in global attributes.
Make two global game attributes, integer type, called PlayerX and PlayerY.
Then, in the Player Actor, add two Constrain Attribute behaviors:
Constrain Attribute: game.PlayerX to: self.Position.X
Constrain Attribute: game.PlayerY to: self.Position.Y
Now, everything in the game has access to the Player's current position, and can act on it.
The next thing I would do is to have a 'range of vision' for the enemies. That way, the enemies only chase the Player is the Player gets close enough to them.
So:
In the Enemy Actor, create a new attribute (integer) called something like 'visionRange' (without the quotes). Set it to 100.
Now we are going to use the magnitude function to determine the distance between the player and the enemy.
Magnitude is a distance calculation between two points. It comes in very handy!
Add this Rule to the Enemy:
Rule
When all conditions are valid:
self.visionRange > magnitude(abs(self.Position.X - game.PlayerX),abs(self.Position.Y - game.PlayerY))
-----Move To: x: PlayerX, y: PlayerY, speed: [whatever]
Now, whenever the Player is inside the Enemy's range of vision, the Enemy will chase the Player.
Hope this helps!
Joe
Either a missing/extra parenthesis, or a 'dangling' attribute.
Make sure you choose ALL attributes from the drop-down menu. Do not just type them in.
I did notice if I set the relative to settings to actor, the games loads then freezes right after a sec.
I don't know what is going wrong. It should work fine.
If you cut out that Rule and place the enemy in the Scene, does it work?
Do you have both of the abs() in the expression as well?
Please check your parentheses again, GS only freezes when there is a syntax error.
Make sure that comma is in there as well...
Copy/Paste what you have in the expression editor in that magnitude field into the forums here, maybe I can see what is going wrong...
Hard to notice those things. Thanks! xD
the abs() functions in the magnitude function are not needed since magnitude is always an absolute value.
@design219
years of coding :P I started when I was 15.. i'm 33 now. (If only I could draw!!!)
finite state machines are just a fancy way of saying that you can change the behavior of an actor based on the conditions of its inputs.
so say you have an actor that switches "modes" between chase and run
each of those two modes is a state, and since there is a finite number of them it's called a "finite state machine"
it allows for very simple creation of semi-dumb AI which changes its behavior based on some in-game critiera... like pack man picking up the power pellet changes the ghosts from "chase" to "run" mode...
you could have any states you can imagin. Chase, run, sleep, eat, fly, explode, invisible, etc..
you just create a self variable called "state" (or whatever you want) then create a rule for each state
Rule: state = "chase"
(firemaple's code here to do the chase stuff)
Rule: state = "run"
(invert firemaple's code and make the value negative to make it run away from the player)
Rule: state "sleep"
(do nothing)
... etc...
that's a very basic finite state machine.
As you can see, this creates the illusion of the AI having a sort of "brain" as it changes its behavior based on the game world's current condition.
@ORBZ: does the magnitude function force a positive? If so, that's great. I didn't know that. I put the abs() in there just in case.
yes magnitude always returns a positive number, or 0. vectorToAngle though can return any real number.
@ FatalCrest
Interesting suggestion... I'd like to learn how to draw simple gfx though in the computer. The problem is, how can I translate paper art to computer art? I have a drawing tablet but what's in my head just does not show up on paper
Oh, wait you knew that!