Variable jump height?
How do you implement a jumping system where the length you hold down the jump button decides how high you jump? i.e a light tap= hop/ long hold= mid-sized jump. This would also mean that the character in the platformer would still have a max jump height, though.
Comments
@Frazzle, it depends on the "feel" you want.
2 easy examples:
Version 1 -
You first check if the jump button was released within let's say <0.1s, this will be a low jump.
If the button is still down after 0.1s then do a high jump.
More realistic jump action, but with slight input lag.
Version 2 -
As soon as the jump button is pressed, start the low jump.
If the button is still pressed after 0.1s, give the actor another boost for the high jump.
Immediate response, but with a "double jump" feel for the high jump.
MESSAGING, X-PLATFORM LEADERBOARDS, OFFLINE-TIMER, ANALYTICS and BACK-END CONTROL for your GameSalad projects
www.APPFORMATIVE.com
Right. So there isn't a way to make the height of the jump directly proportional to the length of time the key is pressed?
@Frazzle, sure there is.
In this case you could force the linear X and Y velocity as long as the jump button is pressed, up to a maximum duration.
MESSAGING, X-PLATFORM LEADERBOARDS, OFFLINE-TIMER, ANALYTICS and BACK-END CONTROL for your GameSalad projects
www.APPFORMATIVE.com
Thanks - i'll try that out.
I've done it, but there's the issue that my character now sticks to the bottom of objects when jumping. Here's the code. (its a bit of a mess) http://frazz3l.tumblr.com/
@Hopscotch
@Frazzle, don't have time to get into your code but can imagine the issue.
You would need to cancel the jump as soon as the character bumps into and object, above or next to it.
Either put invisible objects at the bottom of problem zones and check if the character overlaps/collides with these.
If you have very many potential problem areas like these and you don't want to put invisible colliders everywhere, then constrain small hit-boxes to the top and sides of the character to check for jump breaking collisions.
MESSAGING, X-PLATFORM LEADERBOARDS, OFFLINE-TIMER, ANALYTICS and BACK-END CONTROL for your GameSalad projects
www.APPFORMATIVE.com
Imagined I would have to do this..thanks again mate, @Hopscotch
i too am looking for a few best practises methods for a variable jump height, I have it working but it could be better,
anyways @Frazzle
to detect that the player has indeed hit an actor above and cancel the jump you can do the following, this method doesn't require hitboxes in every actor above.
your main actor "the player" must constrain a world attribute its self Y position.
and also create a world integer attribute that is called cancel jump. this will be either 1 or 0 ie on off
now
within the level tiles have the following code:
if actor overlaps or collides with actor of type/tag "main player"
and
if " self platform actors" Y position is > "greater than" the world attribute of the "main players Y position"
do this:
change attribute: world attribute "cancel jump to "1"
in your main player actor have a rule that is something like, if cancel jump = 1 change self motion velocity to 0 "assuming you have gravity set up within this actor to make it fall back down"
now when the main actor hits the ground you need to switch the world attribute for cancel jump back to 0 when ever the actor is grounded.
basically you can use the same code above to ground your actor too, just change the y position to < "less than" the main character actor.. etc.. if you get me:)
@Hopscotch couldn't you use the high jump low jump method and create a counter within a rule or loop in a separate actor then apply the rule to check for when the button is released in this separate actor and change a global boolean to true to signal the actor player_low_jump.true. Sounds like it should work Im gonna try it out as I need it for my game.
Heres one way to get started doing it
Create an Actor (JumpControls)
create 2 global variable: game.player_Y(Real) game.player_jump(boolean)
Within your player that jumps create 1 self variable: self.player_y(Real)
constrain self.Position.Y to self.player_y
constrain self.player_y to game.player_Y
Now create a rule within your button when touch is pressed change attribute game.player_jump.true
Now with your separate JumpControls actor you can make a rule. When game.player_jump is true and when game.player_Y >orEqual 250
change attribute game.player_jump.false.
You would also have to have other rules within JumpControls to constrain the player_Y player_Y+2 or something when game.player_jump.true and another to reduce the Y axis when game.player_jump.false
See attached for example to answer your question in one way for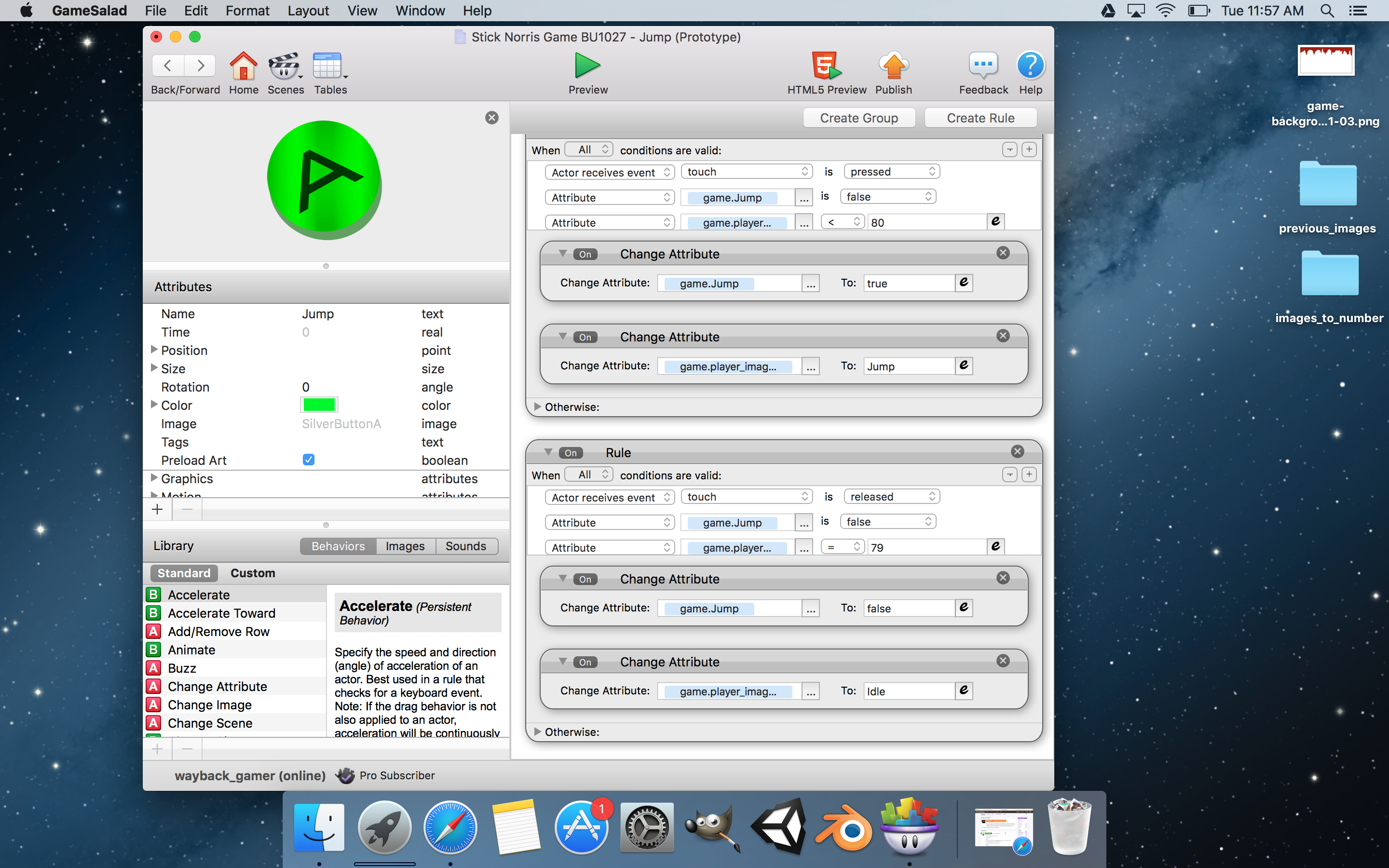
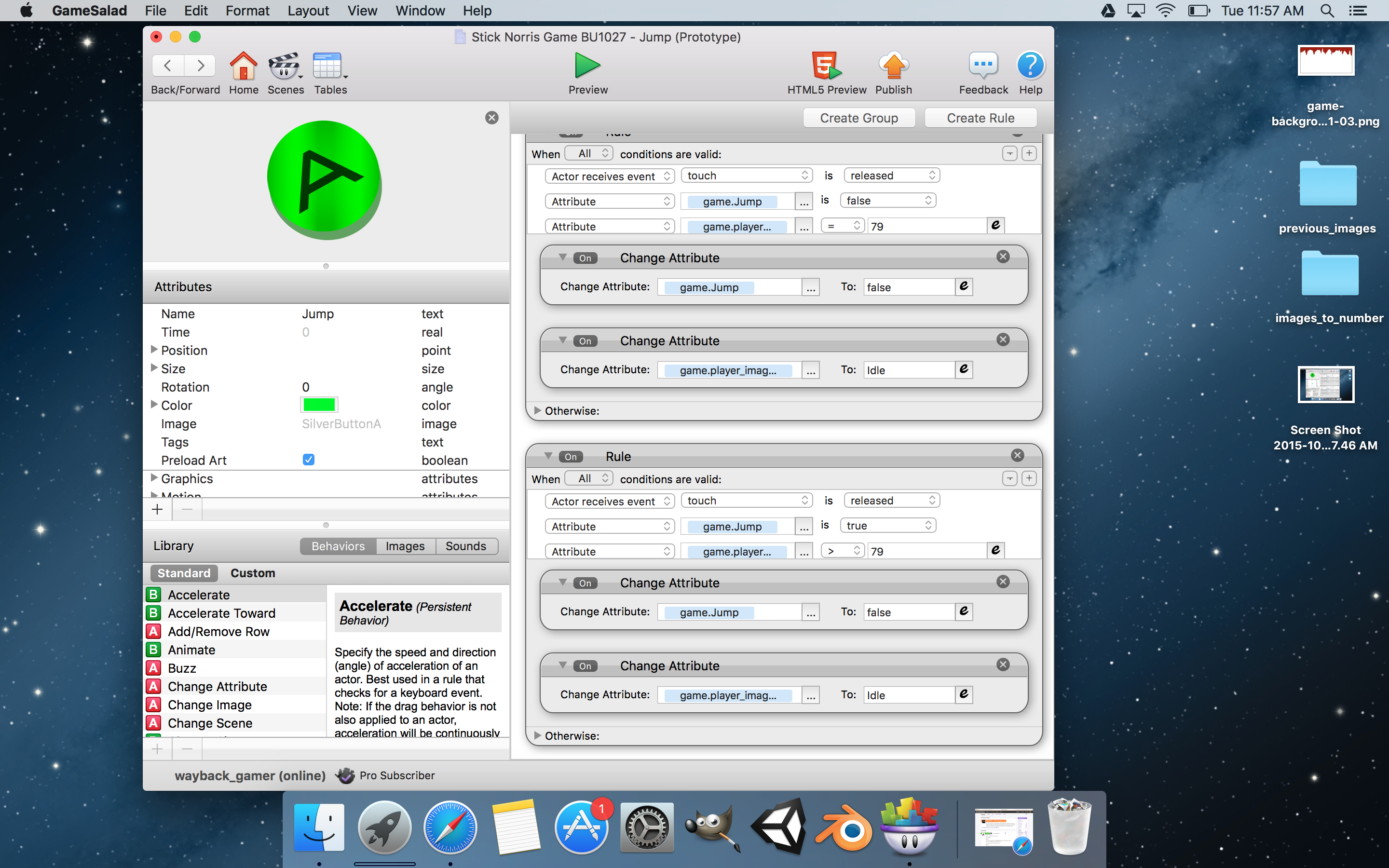
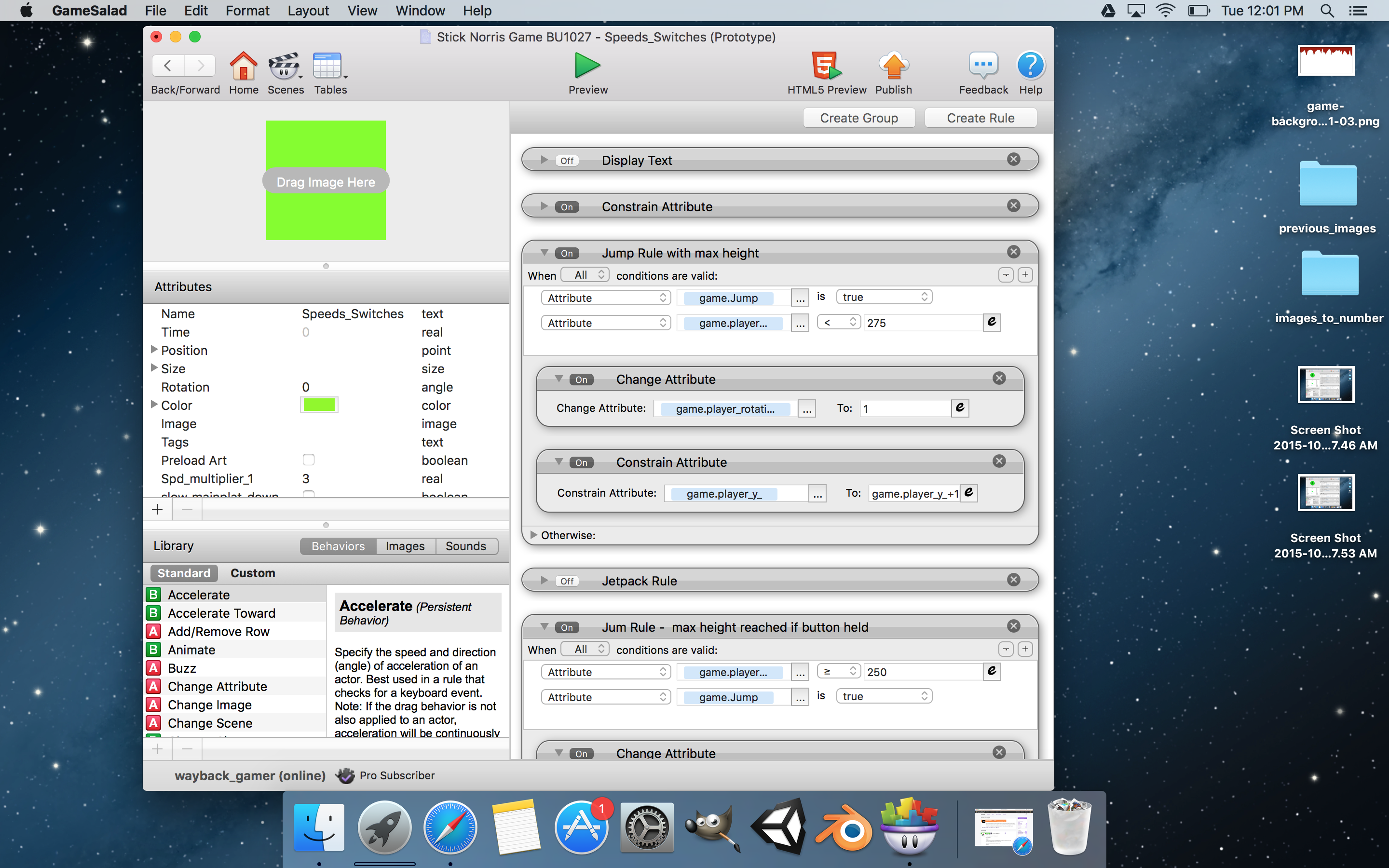
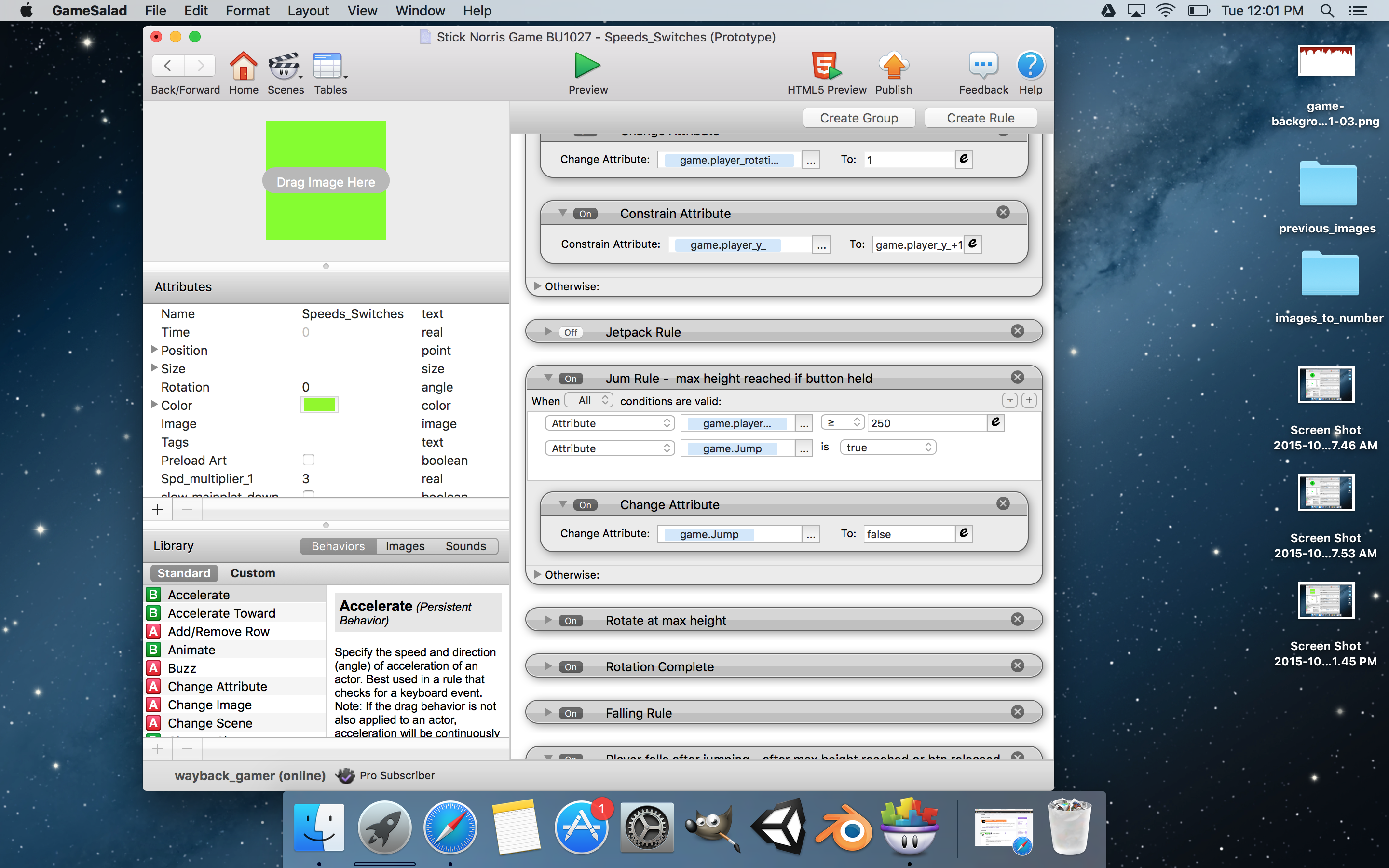
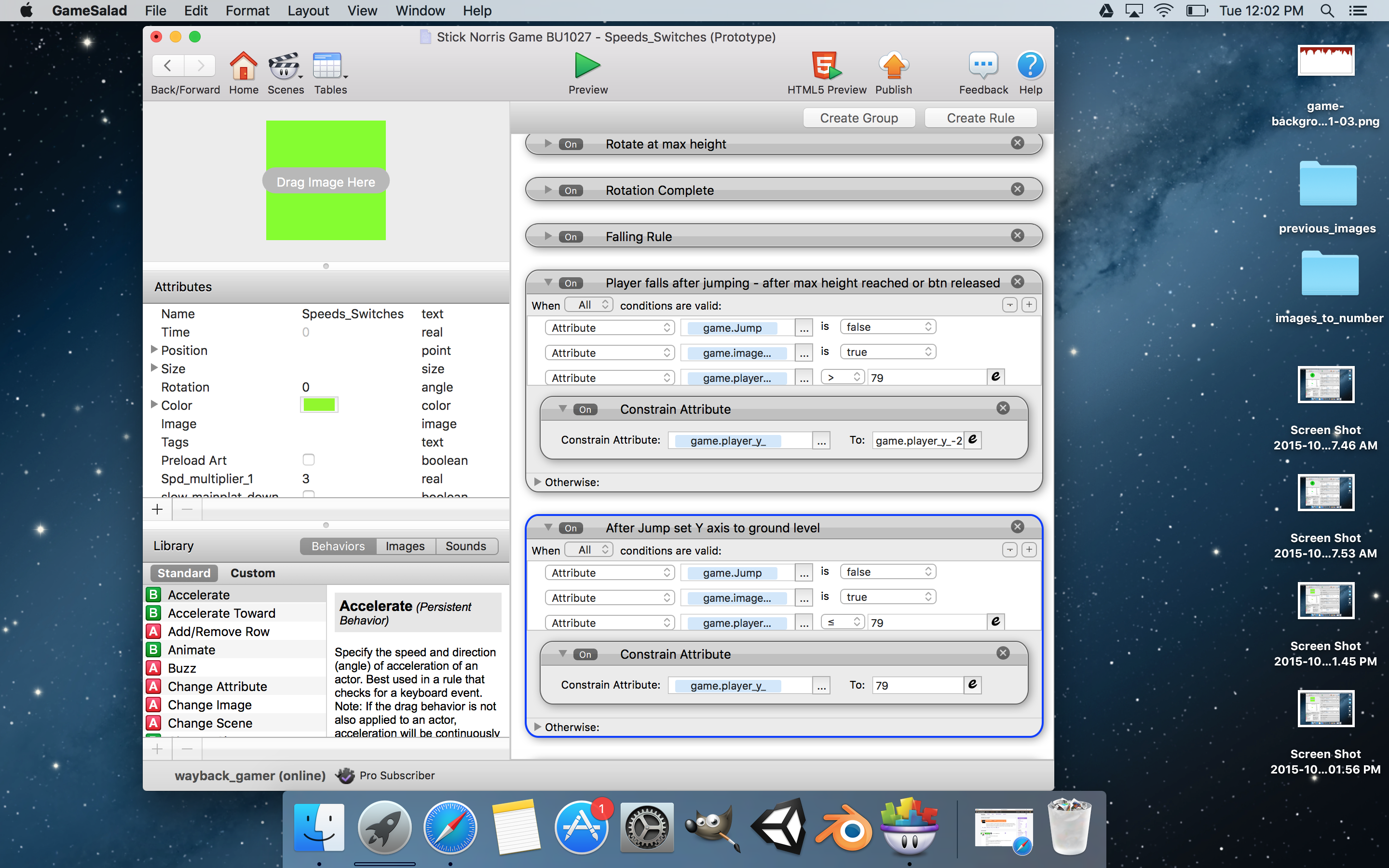
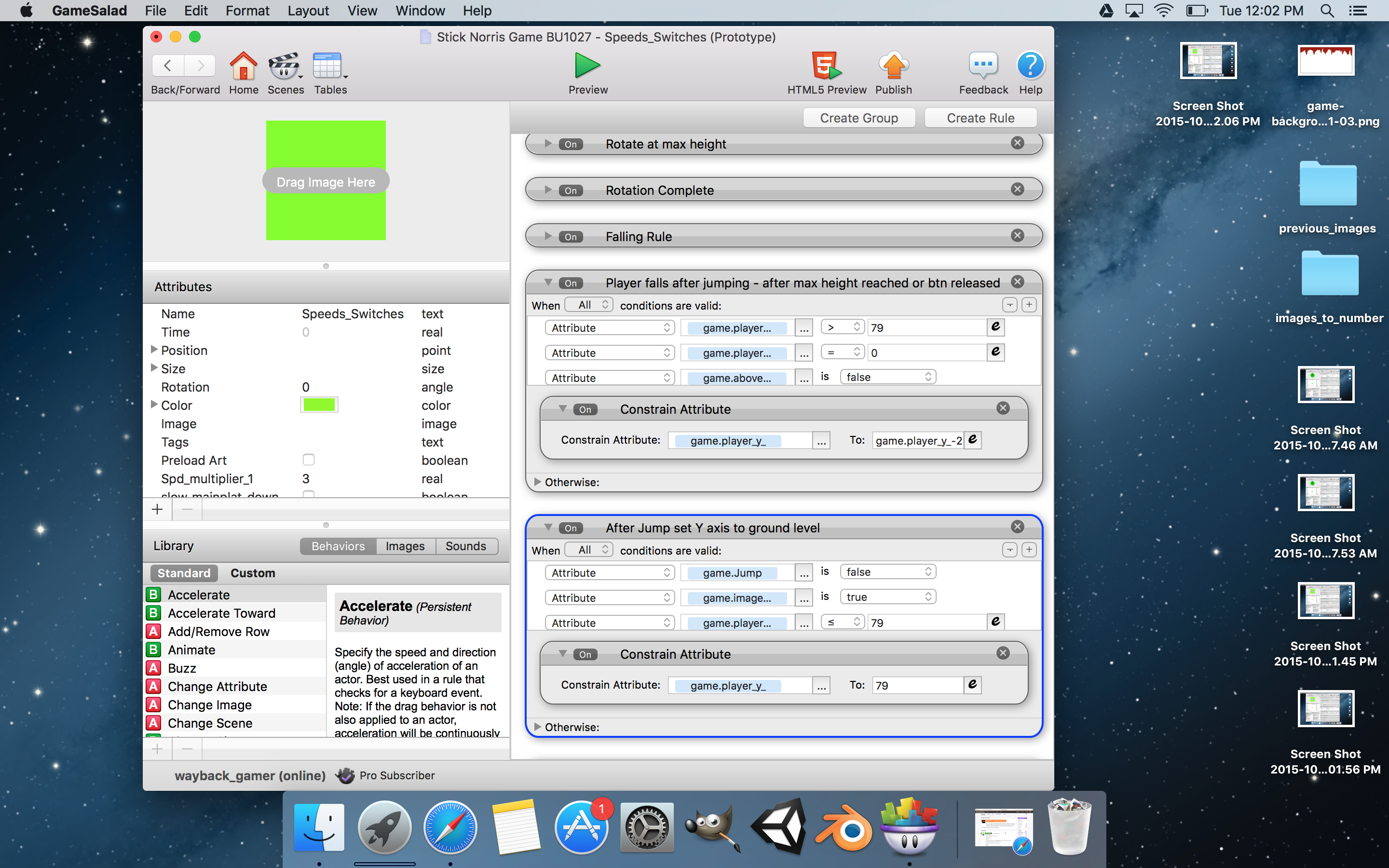
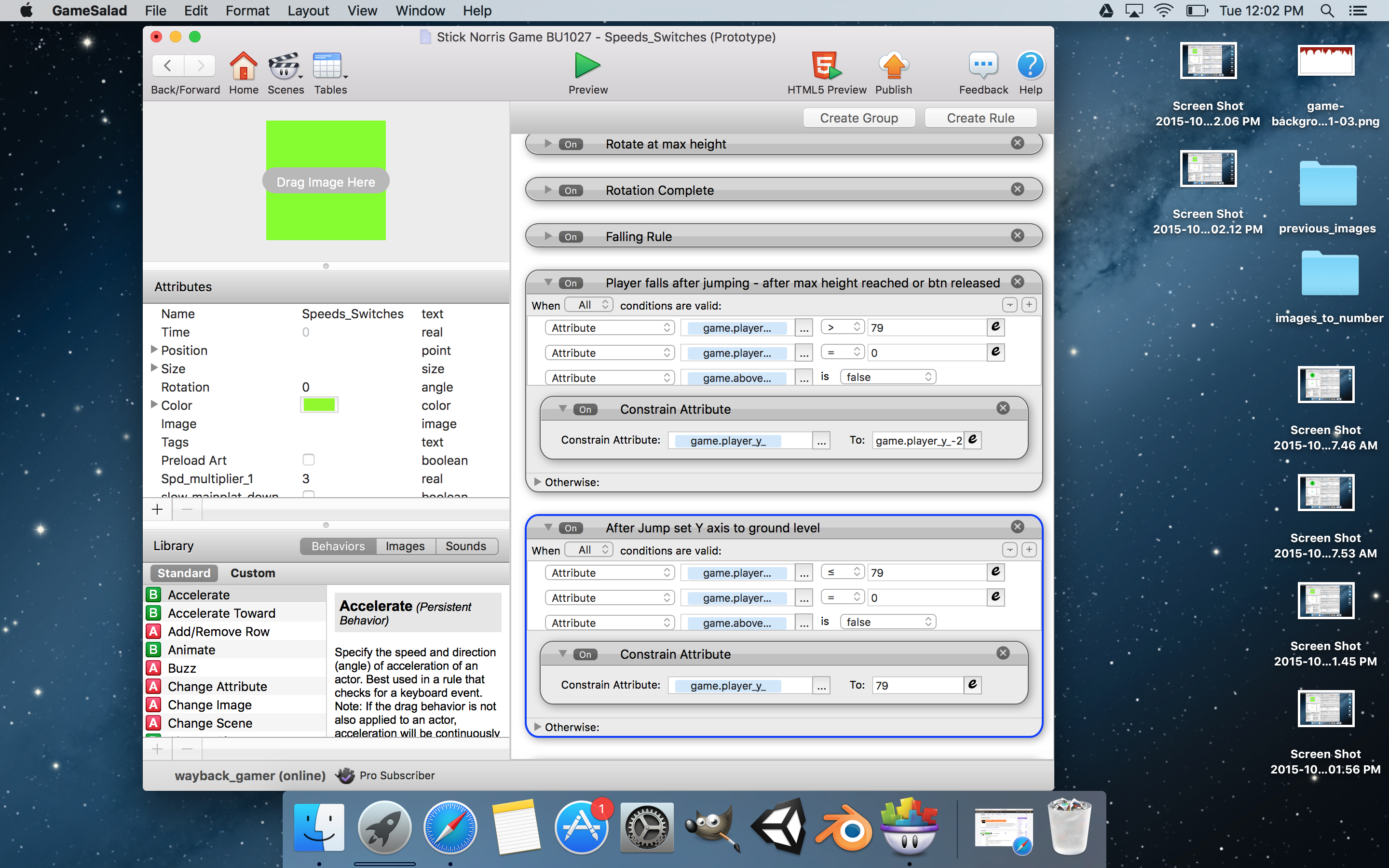
variable jump height.